[Maya] get adjacent vertices to a vertex
MEL script to get a vertices directly connected to another vertex. - 02/2024 - #Jumble
Simple scripts to get the first ring of neighborhood of a vertex of a mesh in Maya.
MEL procedures
string $selectedVertices[] = `ls -selection -flatten`; // Convert the selected vertices to vertex faces string $vertexFaces[] = `polyListComponentConversion -fromVertex -toVertexFace $selectedVertices`; // Convert the vertex faces to vertices (this will give adjacent vertices) string $adjacentEdges[] = `polyListComponentConversion -fromVertexFace -toEdge $vertexFaces`; // Convert the edges to vertices (this will give adjacent vertices) string $adjacentVertices[] = `polyListComponentConversion -fromEdge -toVertex $adjacentEdges`; $adjacentVertices = `ls -flatten $adjacentVertices`; //select $adjacentVertices; // Filter out the original selected vertices from the adjacent vertices list string $adjacentVerticesFiltered[] = `stringArrayRemove $selectedVertices $adjacentVertices`; select $adjacentVerticesFiltered;
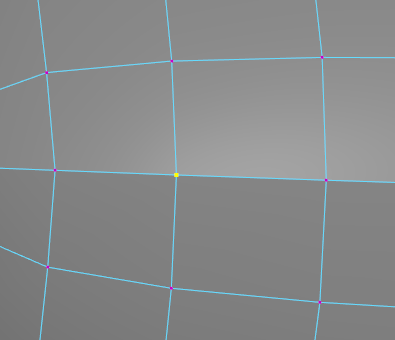
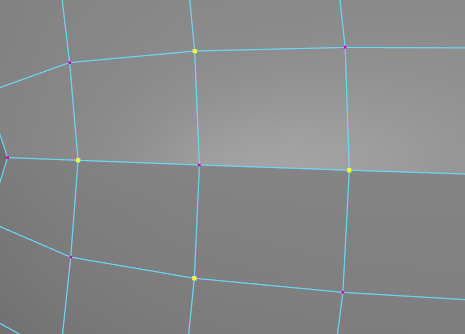
Less conservative version:
string $selectedVertices[] = `ls -selection -flatten`; // Convert the selected vertices to vertex faces string $faces[] = `polyListComponentConversion -fromVertex -toFace $selectedVertices`; // Convert the edges to vertices (this will give adjacent vertices) string $adjacentVertices[] = `polyListComponentConversion -fromFace -toVertex $faces`; $adjacentVertices = `ls -flatten $adjacentVertices`; //select $adjacentVertices; // Filter out the original selected vertices from the adjacent vertices list string $adjacentVerticesFiltered[] = `stringArrayRemove $selectedVertices $adjacentVertices`; select $adjacentVerticesFiltered;
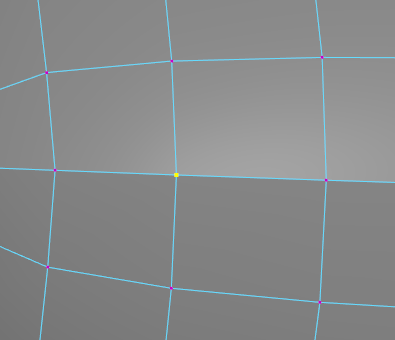
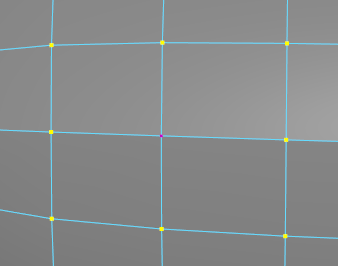
Python
import maya.api.OpenMaya as om from maya import cmds obj_name = "pCube1" def get_connected_verts(obj_name, vert_index): vit = om.MItMeshVertex(get_shape(obj_name)) vit.setIndex(vert_index) res = vit.getConnectedVertices() return res vertices = get_connected_verts(obj_name, 431) vertex_names = ["{}.vtx[{}]".format("pCube1", index) for index in vertices] cmds.select(vertex_names, replace=True) # Or: select_vertices(obj_name, vertices)
def select_vertices(obj_name:str, vert_list:om.MIntArray) : mfn_components = om.MFnSingleIndexedComponent(get_MObject(obj_name)) mfn_object = mfn_components.create(om.MFn.kMeshVertComponent) mfn_components.addElements(vert_list) selection_list = om.MSelectionList() tuple = (get_MDagPath(obj_name), mfn_object) selection_list.add(tuple) om.MGlobal.setActiveSelectionList(selection_list) def get_MDagPath(obj): if isinstance(obj, om.MDagPath): return obj if not isinstance(obj, om.MObject): obj = get_MObject(obj) assert obj.hasFn(om.MFn.kDagNode), ("This MObject is not a valid dag node, current type: "+obj.apiTypeStr()) return om.MFnDagNode(obj).getPath() def get_MObject(node_name): if isinstance(node_name, om.MObject): return node_name if isinstance(node_name, om.MDagPath): return node_name.node() slist = om.MSelectionList() try: slist.add(node_name) except RuntimeError: assert False, 'The node: "'+node_name+'" could not be found.' matches = slist.length() assert (matches == 1), 'Multiple nodes found for the same name: '+node_name obj = slist.getDependNode(0) return obj def get_shape(node_name): if isinstance(node_name, om.MDagPath): dag_path = node_name dag_path.extendToShape() return dag_path.node() if isinstance(node_name, om.MObject): node = node_name elif isinstance(node_name, str): node = get_MObject(node_name) else: assert False, "unexpected type" # Find a shape node directly underneath the node. dag_path = om.MDagPath() dag_path = get_MDagPath(node) dag_path.extendToShape() return dag_path.node()
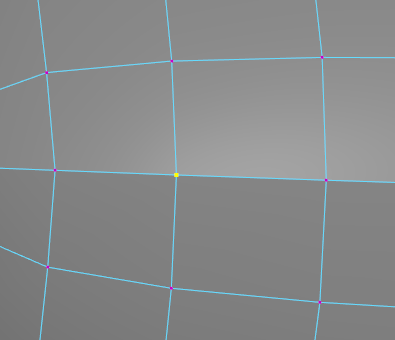
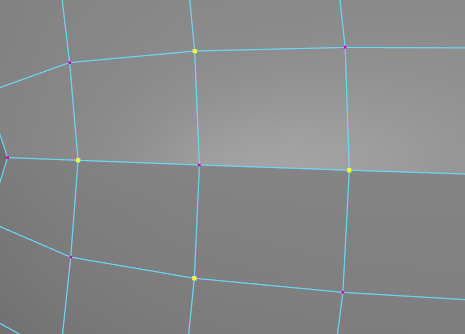
No comments